What is Drush?
Drush is a tool you use in the command line to manage Drupal websites. It comes with many helpful commands to do things like updating your site, running SQL queries, migrating content, and handling tasks like running cron jobs or clearing the cache. You can also add more commands to Drush by using third-party extensions.
Basic Drush commands :-
drush status
- Description: Displays the current status of your Drupal site.
- Example:
drush status
- Output: Information about the Drupal version, database connection, PHP version, and other important details.
drush cr
- Description: Clears all cached data.
- Example:
drush cr
- Output: Clears the cache, helping to refresh and rebuild the site’s data.
drush en [module_name]
- Description: Enables a specified module.
- Example:
drush en views
- Output: Enables the "Views" module on your Drupal site.
drush pmu [module_name]
- Description: Uninstalls a specified module.
- Example:
drush pmu views
- Output: Uninstalls the "Views" module from your Drupal site.
drush updb
- Description: Runs database updates.
- Example:
drush updb
- Output: Applies any pending database updates needed after a module or core update.
drush uli
- Description: Generates a one-time login link for the admin user.
- Example:
drush uli
- Output: A URL you can use to log in to your Drupal site as the admin without needing a password.
drush cim
- Description: Imports configuration changes.
- Example:
drush cim
- Output: Imports configuration from YAML files into the active configuration storage.
drush cex
- Description: Exports the current configuration to YAML files.
- Example:
drush cex
- Output: Exports the site's configuration to files, which can be version controlled.
drush sql-dump
- Description: Dumps the Drupal database to a SQL file.
- Example:
drush sql-dump > backup.sql
- Output: Creates a backup of the database in a file named
backup.sql
.
drush sql-cli
- Description: Opens an SQL command-line interface connected to the Drupal database.
- Example:
drush sql-cli
- Output: Provides direct access to the database, allowing you to run SQL commands.
drush migrate:import [migration_id]
- Description: Runs a specified migration.
- Example:
drush migrate:import my_migration
- Output: Executes the migration process for the specified migration ID.
drush cron
- Description: Runs all cron hooks in the Drupal site.
- Example:
drush cron
- Output: Executes scheduled tasks, such as clearing old logs and running periodic updates.
Create custom drush command
There are two steps to create the custom drush command using custom module which are as follows
- Manual process
- Automatic process (Using Drush)
Manual Process :-
- Create the new custom module or you can also use the existing custom module and create the directory
src
inside the module root directory. - Create the
Drush
directory inside thesrc
directory. - Create the
Commands
directory inside theDrush
directory. - Inside the
Commands
directory, create a PHP file for your drush command e.g.CustomDrushCommand.php
- Extend the
CustomDrushCommand
class viaDrushCommands
class. - Here is the module directory structure.
web/modules/custom/custom_module src |--- Drush |------ Commands |--------- CustomDrushCommand.php custom_module.info.yml custom_module.module
Here is the content of the
CustomDrushCommand.php
file<?php namespace Drupal\custom_module\Drush\Commands; use Drush\Commands\DrushCommands; /** * Custom Drush command */ class CustomDrushCommand extends DrushCommands { /** * Custom Drush command. * * @command custom_module:hello-world * @aliases mch * @description Prints "Hello, World!" message. */ public function hello() { $this->output()->writeln('Hello, World!'); } }
- Clear the cache and test the custom command.
- Run
drush custom_module:hello-world
and you will get the output asHello, World!
Automatic Process (using Drush)
- Run the
drush generate
command and you will see the list of all available commands.
Image
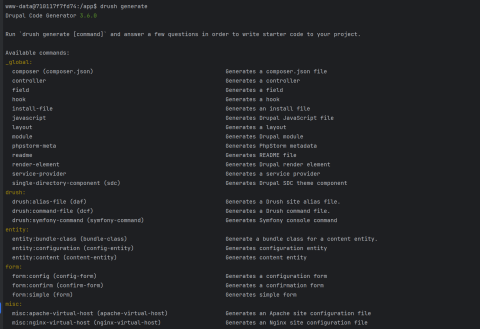
- Now run the
drush generate drush:command-file
ordrush generate dcf
command.
Image
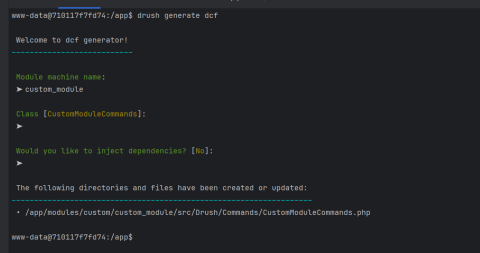
- Now your file is created with the name
CustomModuleCommands.php
without manually creating the directories and file. - It is currently not creating the content inside the
CustomModuleCommands.php
file but it might create some demo command in future. - Edit the
CustomModuleCommands.php
file and add the following content.
<?php
namespace Drupal\custom_module\Drush\Commands;
use Drush\Commands\DrushCommands;
/**
* Custom Drush command
*/
class CustomModuleCommands extends DrushCommands {
/**
* Custom Drush command.
*
* @command custom_module:hello-world
* @aliases mch
* @description Prints "Hello, World!" message.
*/
public function hello() {
$this->output()->writeln('Hello, World!');
}
/**
* Custom Drush command.
*
* @command custom_module:hello-world2
* @aliases mch
* @description Prints "Hello, World 2!" message.
*/
public function hello2() {
$this->output()->writeln('Hello, World 2!');
}
}
- You can add multiple command with multiple functions in the same file see the above php file.